
30 Python Programming Questions on Operators and Expressions
Q 1. Write a Python program to add two numbers.
Input: a = 10, b = 5
Expected Output
15
Q 2. Write a Python program to subtract one number from another.
Input: a = 15, b = 8
Expected Output:
7
Q 3. Write a Python program to multiply two numbers.
Input: a = 4, b = 6
Expected Output:
24
Q 4. Write a Python program to divide one number by another.
Input: a = 20, b = 4
Expected Output:
5.0
Q 5. Write a Python program to find the remainder when one number is divided by another.
Input: a = 15, b = 4
Expected Output:
3
Q 6. Write a Python program to perform floor division of two numbers.
Input: a = 17, b = 3
Expected Output:
5
Q 7. Write a Python program to calculate the power of a number.
Input: a = 2, b = 3
Expected Output:
8
Q 8. Write a Python program to check if a number is greater than another.
Input: a = 10, b = 7
Expected Output:
True
Q 9. Write a Python program to check if a number is less than another.
Input: a = 3, b = 9
Expected Output:
True
Q 10. Write a Python program to check if two numbers are equal.
Input: a = 5, b = 5
Expected Output:
True
Q 11. Write a Python program to check if two numbers are not equal.
Input: a = 4, b = 10
Expected Output:
True
Q 12. Write a Python program to check if a number is greater than or equal to another.
Input: a = 7, b = 7
Expected Output:
True
Q 13. Write a Python program to check if a number is less than or equal to another.
Input: a = 4, b = 9
Expected Output:
True
Q 14. Write a Python program to perform logical AND operation.
Input: a = True, b = False
Expected Output:
False
Q 15. Write a Python program to perform logical OR operation.
Input: a = True, b = False
Expected Output:
True
Q 16. Write a Python program to perform logical NOT operation.
Input: a = True
Expected Output:
False
Q 17. Write a Python program to perform bitwise AND operation.
Input
a = 5 # 0101
b = 3 # 0011
Expected Output:
1
Q 18. Write a Python program to perform bitwise OR operation.
Input:
a = 5 # 0101
b = 3 # 0011
Expected Output:
7
Q 19. Write a Python program to perform bitwise XOR operation.
Input
a = 5 # 0101
b = 3 # 0011
Expected Output:
6
Q 20. Write a Python program to perform bitwise NOT operation.
Input: a = 5 # 0101
Expected Output:
-6
Q 21. Write a Python program to perform left shift operation.
Input: a = 5 # 0101
Expected Output:
10
Q 22. Write a Python program to perform right shift operation.
Input: a = 5 # 0101
Expected Output:
2
Q 23. Write a Python program to calculate the result of an arithmetic expression with parentheses.
Input: a = 10, b = 2, c = 3
Expected Output:
36
Q 24. Write a Python program to use the exponentiation operator in an expression.
Input: a = 2, b = 3, c = 2
Expected Output:
10
Q 25. Write a Python program to use the modulus operator in an arithmetic expression.
Input: a = 14, b = 4
Expected Output:
5
Q 26. Write a Python program to combine addition, multiplication, and division in an expression.
Input: a = 20, b = 4, c = 3
Expected Output:
26.0
Q 27. Write a Python program to use the comparison operator to check if a number is within a range.
Q 28. Write a Python program to use the assignment operator with addition.
Input: a = 5, a += 3
Expected Output:
8
Q 29. Write a Python program to use the assignment operator with subtraction.
Input: a = 10, a -= 2
Expected Output:
8
Q 30. Write a Python program to use the assignment operator with multiplication.
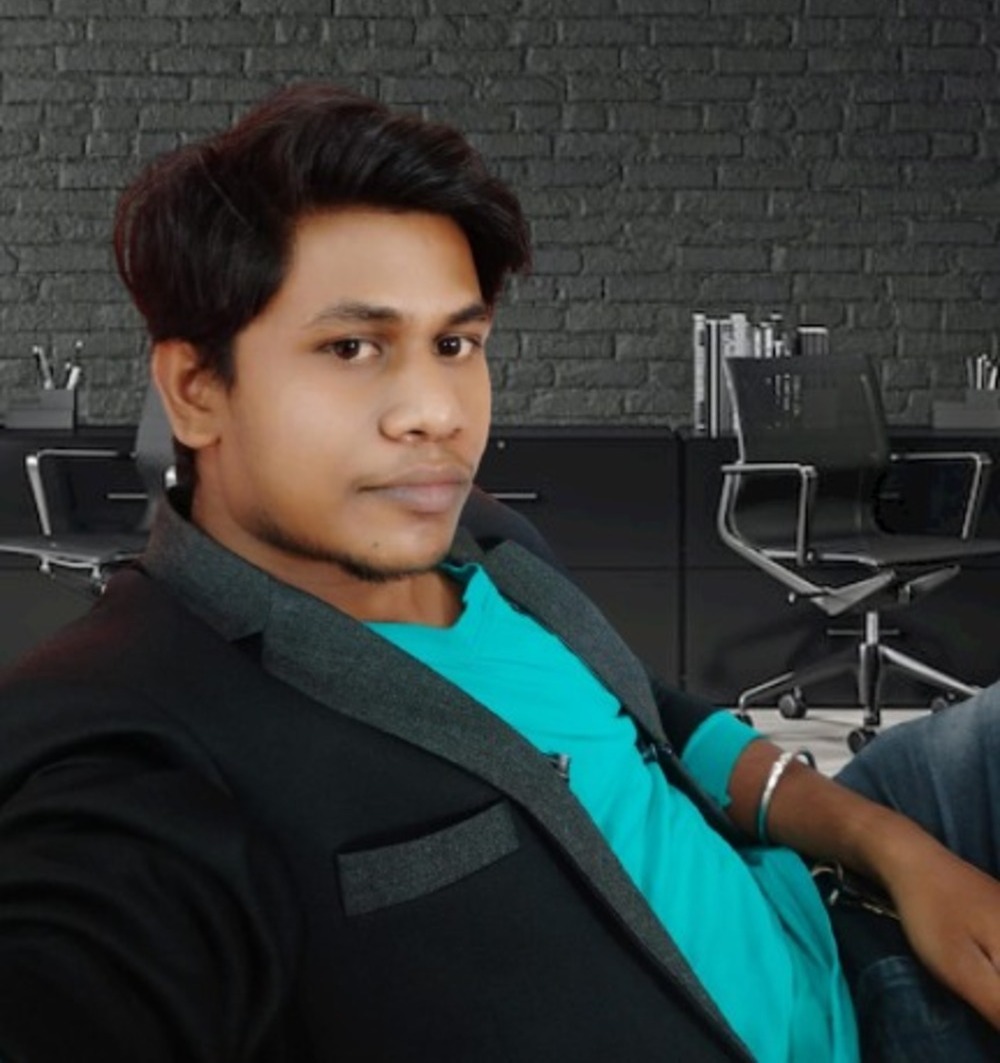
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!